Managing lists (or arrays as known in other languages) in python is a common task where you often need to take a section from the list – e.g. a slice of the list. This is where slice() can be used to take a section of the list. There are several ways to do this, read on to find out more.
In this article you will start with the basics of the slice() function. You will learn about the data types supported by slice() function and the parameters required by slice() function. Further you will learn with example how to extract sub-strings from a string, how to extract a sub-list or sub-tuple from a list or a tuple. You will also learn about an alternative to slice to extract sub-strings.

After you have gone through the fundamentals and practised the example with confidence, there are many real world problems where you can use slice().
Getting started with slice()
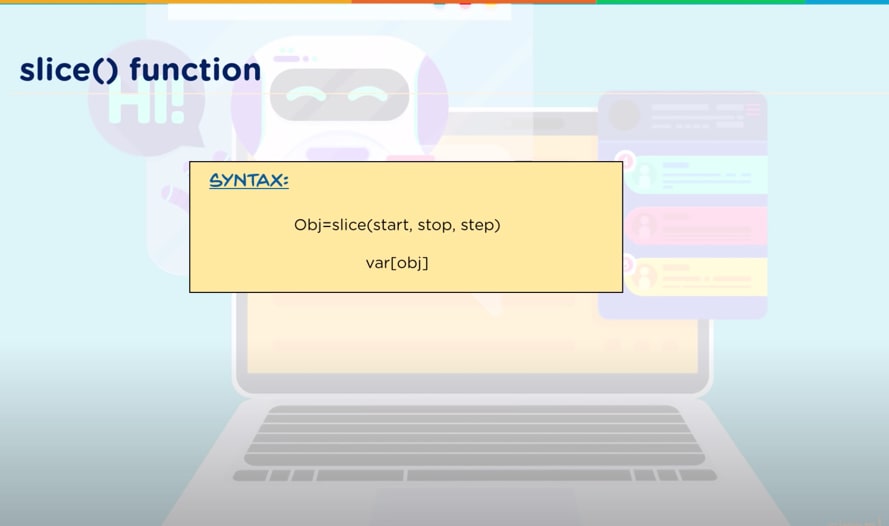
If you take the idea of a loaf of bread, you never eat the whole loaf in one go, you would either cut it into individual slices or take a portion of the bread. slice() function in python exactly does the same. It is a built-in function that returns a section of the object. Slice may be used to extract sub-string, sub-lists, or sub-tuples from a given string, lists, or tuples.
Data Types supported by slice()

Data types which are sequence of objects are supported by slice(). Such data types are string, list, tuple, range, and bytes. Along with data types following the sequence protocol, objects that uses dunder methods like _ _getitem_ _() and _ _len_ _() can also be sliced using slice().
NOTE: Dunder methods is a core python feature, rare in other programing languages. It is used to emulate the functionality of built-in types to a user-defined object. The name comes from double underscores used in suffix and prefix of the methods. It is also called “magic method”.
Parameters required by slice()
The parameters that are required by slice() function are generally called slice notation. They are start, stop, and step.
- start: An integer index value which represents from where the slicing of the object starts. It is an optional parameter.
- stop: An integer index value which represents from where the slicing of the object ends. The element at this index is excluded from the slice. It is mandatory parameter.
- step: An integer value indicating the number of steps(indices) skip while traversing from start index to stop index. It is also an optional parameter.
NOTE: Optional parameters like start and step are defaulted to when those value are not provided.
SYNTAX
slice(stop)
slice(start, stop, step)
Executing the above syntaxes returns a sliced object which is in the range of the parameters provided. Let us learn this with an example.
Example 1: Creating the slice object
# [Insert to example1.py file]
# Providing only stop as the argument
object1 = slice(4)
print(object1)
print(type(object1))
# Providing values to all slice notations.
object2 = slice(1, 6, 2)
print(object2)
print(type(object2))
OUTPUT

From the output you can see that object1 and object2 are objects of slice class, or simply slice objects.
From the above example you might have been able to create a slice object. Further you will learn how to use the slice object to extract sub-strings, sub-list, or sub-tuple from given string, list, or tuple.
Example 2: Using slice object to extract substring
String is the most common data type used in Python. It is used to store characters. Each character in a string data type is indexed. As such, each characters can be individually accesses using its index value.

In the above figure you can view a word ‘BANANA’. In Python it can be assigned to a variable, making it a string type variable. When used len() function the result for this string will be 6. Simply, the total number of characters or letters present in the word ‘BANANA’. len() function is used to calculate the length of a string or the number of characters in a string. Empty space is also considered as a character.
Index is simply an address in the memory location. Whenever a variable is created a memory is allocated in the working memory of a device. Using the indexes you can access each characters. For example if the word ‘BANANA’ is stored in a variable named Fruit, then Fruit[1] will result in ‘A’.
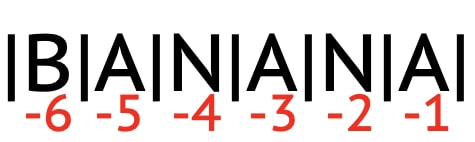
Indexing in python goes both ways either positive or negative. You can access characters even using the negative indexes as the figure above. Negative indexing is useful when you aren’t known about the total length of the character and want to quickly access the last character.
# [Insert to example2.py file]
# Extract substring from a given string
website = 'pythonhowtoprogram.com'
# stop = -4
# Using negative index
slice_object = slice(-4)
print(website[slice_object])
# start = 1, stop = 6, step = 2
# Using positive index
slice_object = slice(1, 6, 2)
print(website[slice_object])
OUTPUT

Example 3: Using slice object to extract sub-list
List is collection type data-type. It allows you to place multiple values or item in a single variable. Such values that are inserted in a list are called list constants. A list is surrounded by square brackets and list elements or list constants are separated by commas. List is a mutable data-type. It means that elements within the list can be changed. Each element of list has individual indexes, similar to the string indexes mentioned above. You can access the elements using the indexes.
Elements in the list are ordered. When new element is added in a list, it is added to the end of the list.
# [Insert to example3.py file]
list1 = ['P', 'y', 't', 'h', 'o', 'n','h','o','w']
# Using Positive index
# stop = 3
slice_object = slice(3)
print(list1[slice_object])

# Using Negative indexes
# start = -1, stop = -5, step = -2
slice_object = slice(-1, -5, -2)
print(list1[slice_object])

Using Indices Notation
There is also a more straightforward way to use slices which avoids creating or using the slice object which is to use the square bracket notation. However, you cannot uses steps in this form. This should still help in most of your scenarios.
The idea is to use [<start>:<end>] notation. The start and end number can be either positive or negative – much like slice. Here are several teamples
a = [22, 44, 55, 66, 77, 88]
print( a[0:4] ) #Print the first 5 items
print( a[:4] ) #Print the first 5 items - 0 is default
print( a[1:] ) #Print all skipping item 1
print( a[-1:] ) #Print just the last element
print( a[-2:] ) #Print the last 2 elements
print( a[:-1] ) #Print all items excluding last item

Subscribe to our newsletter
Error SendFox Connection: {“message”:”Unauthenticated.”}